In our previous tutorial, we have learnt about the core part of J2ME applications, i.e. MIDlet. In this tutorial, we will be learning about using different User Interfaces, and how to handle the user interactions with your applications (handling the events).
Two basic packages for User Interactions are:
- javax.microedition.lcdui.*;
- javax.microedition.lcdui.game.*;
First package defines user controls, event handling mechanism and graphical components for mobile applications. Latter one extends the features of the lcdui components such as Canvas , Image etc to provide more game specific features such as small animations. Read the API documentation for more details about the packages here.
In this tutorial, we will simply create a list menu, then a form where we will be using different UI Components, and then add commands (which act like buttons), and finally demonstrate the event handling for the above components.
The list of UI Components that will be demonstrated in this tutorial:
span class="small">javax.microedition.lcdui.Form
javax.microedition.lcdui.List
javax.microedition.lcdui.TextField
javax.microedition.lcdui.ChoiceGroup
javax.microedition.lcdui.Command
Let us define the attributes which would be used in MyFirstApp MIDlet:
private Form form;
private List mainmenu;
private Display display;
public TextField txtField;
public ChoiceGroup cGroup;
public Command backCommand;
public Command okCommand;
First up, we will be creating a list menu which would be displayed as soon as the MIDlet is loaded. We will be using List to create such interface.
Constructor used to create a List object is as follows:
public List(String title,int type)
We can define different types of List using static attributes of Choice such as:
- Choice.IMPLICIT : To create list associated with SELECT_COMMAND, where an event is generated once the option is selected which can be handled by using CommandListener Interface
- Choice.EXCLUSIVE: To create list with exclusive selection option i.e. Radio buttons
- Choice.MULTIPLE: To create list with multiple selection option i.e. Check Boxes
Note: Choice.EXCLUSIVE and Choice.MULTIPLE don’t generate any events. Method used for adding items or options to the list is:
public void append(String stringPart, Image imgpart )For this tutorial we would be creating list of IMPLICIT type as we want to act the options of the list to act as Menu so that we can trigger an event handling code once the user selects the list option. Following code demonstrates how to create such list and set it as the current MIDlet display:
public Displayable getMainMenu() // Create our main menu {
mainmenu =new List(“Main Menu”,Choice.IMPLICIT);
mainmenu.append(“Open Demo”, null);
mainmenu.append(“Exit”, null);
mainmenu.setCommandListener(comListener); // Registering Listener for the List Component
return mainmenu;
}
public void startApp() {
display = Display.getDisplay(this); // gets the display component of MIDlet class display.setCurrent(this.getMainMenu()); //sets the above created menu as the current display
} //end of StartApp
Menu we just created will be displayed as follows once we run the application:
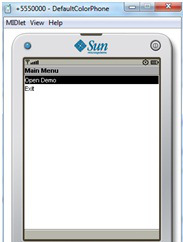
Now lets create our next displayable component Form and add more components to that Form such as TextField, ChoiceGroup and Commands.
Constructor used to create Form is as follows:
public Form(String title)
Create TextField using following constructor:
public TextField(String label,String value,int size, int constraints)
where in constraints parameters, several static TextField constraint values are used such as TextField.ANY, TextField.PASSWORD, TextField.NUMERIC, TextField.UNEDITABLE etc.
Create ChoiceGroup using Following constructor:
public ChoiceGroup(String title,int type)
where type parameter is to specify whether this choicegroup is exclusive i.e. radiobutton or multiple choicegroup i.e. checkbox
Create Commands using following constructor:
public Command(String label,int type, int priority)
where command type is used to specify different command types such as BACK,OK,CANCEL,EXIT,HELP etc…
Form functions to add above components into the form:
public void append(Item itmObj)
public void addCommand(Command cmdObj)
After learning about the above components, now let us create the form and include above components using following code:
public Displayable getForm()
{ //creating a form with textfield, textbox, radio buttons
form=new Form(“My Demo Form”);
//creating blank textfield with label Name of size 30
txtField=new TextField(“Name”,”",30,TextField.ANY);
//Creating exclusive choicegroup with label Gender
cGroup=new ChoiceGroup(“Gender”,ChoiceGroup.EXCLUSIVE);
//Appending items to the choicegroup male and female
cGroup.append(“Male”,null);
cGroup.append(“Female”,null);
//creating command to navigate back
backCommand=new Command(“Go Back”,Command.BACK,2);
//creating command to process form
okCommand=new Command(“DONE”,Command.OK,1);
//adding commands to form
form.addCommand(backCommand);
form.addCommand(okCommand);
//adding form elements to form
form.append(txtField);
form.append(cGroup);
return form;
}
//to view the form
public void startApp() {
display = Display.getDisplay(this); // gets the display component of MIDlet class display.setCurrent(this.getForm()); //sets the above created form as the current display
} //end of StartApp
Now, form we created will be displayed as follows once we run the application:
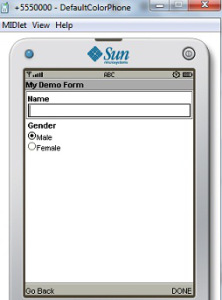